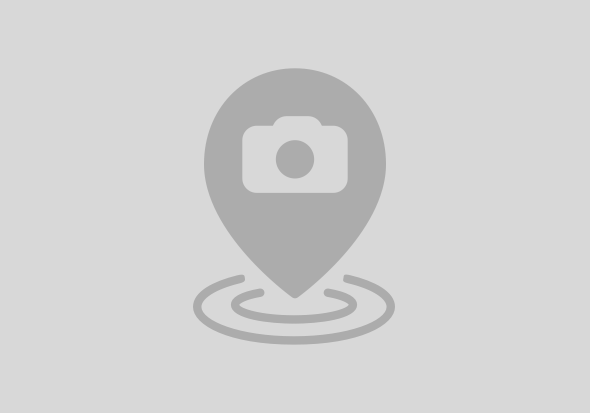
git clone https://github.com/SouravDas25/cloud-foundry-local-setup.git
- account-info.json # contains the cf account information, e.g. org, space, API-endpoint
- accounts-env # contains the downloaded CF environments
- app-router # contains local app router configurations
- apps.json # contains a list of applications present in the cf environments
- deps # static dependency for local app setup, e.g. Local Tomcat
- env.json # contains local app setup settings, e.g. cf account, Shell
- package.json # contains an npm dependency script to run different apps & app router
- scripts # contains local setup scripts
npm install
{
"default-account": {
"org": "sampleorg", //update your cf org
"space": "samplespace", // update your cf space
"apiEndpoint": "https://api.cf.hana.ondemand.com", // API endpoint of cf account
"subdomain": "sampleorg" //account subdomain, use consumer subdomain for consumer account login
}
}
{
"activeAccount": "default-account",
"shell": {
"useCustom": false
}
}
Dependency: CF CLI should be installed.
npm run cf-login
> cloud-foundry-local-setup % npm run cf-login
> cf-login
> cd scripts && node cf-login.js
cf login -o mytrial -s dev --sso -a https://api.cf.us10-001.hana.ondemand.com
API endpoint: https://api.cf.us10-001.hana.ondemand.com
Temporary Authentication Code ( Get one at https://login.cf.us10-001.hana.ondemand.com/passcode 😞
Authenticating...
OK
Targeted org mytrial
Targeted space dev
API endpoint: https://api.cf.us10-001.hana.ondemand.com (API version: 3.140.0)
User: sourav.das01@sap.com
Org: mytrial
Space: dev
i353584@C02Z10RELVDQ cloud-foundry-local-setup %
Note: If you don't have a ready application(s), a set of sample applications has been provided in the 'deps' folder. Build and deploy the 'sample-java' and 'sample-ar' MTARs.
{
"sample-java": {
"cfAppName": "sample-java", // your cf application name
"folder": "./deps/sample-java", // local dir of the spring boot app
"path": "sample-module/target", // path to war file
"artifactName": "sample-java.war", // war file name
"build:cmd": "mvn clean install", // build command
"start:cmd": "-Denvironment=cf -Dspring.profiles.active=provider,cloud -Xdebug -Xrunjdwp:server=y,transport=dt_socket,address=8000,suspend=n", // add profiles for tomcat start
"env": {}
}
}
{
"app-router": {
"cfAppName": "sample-ar", // only update the cf app-router name
"folder": "app-router", //don't modify this,
"env": {
}
},
}
npm run pull-env
> cloud-foundry-local-setup % npm run pull-env
> pull-env
> cd scripts && node pull-env.js
==> getting cf oauth-token
bearer ********
==> Org mytrial
==> Space dev
==> fetching cf org mytrial --guid
==> fetching cf space dev --guid
==> fetching cf app sample-ar --guid
==> downloading cf env sample-ar
==> persisting sample-ar environment.
==> fetching cf app sample-java --guid
==> downloading cf env sample-java
==> persisting sample-java environment.
==> updating account-info file.
{
"sample-java": {
"cfAppName": "sample-java", // your cf application name
"folder": "./deps/sample-java", // local dir of the spring boot app
"path": "sample-module/target", // path to war file
"artifactName": "sample-java.war", // war file name
"build:cmd": "mvn clean install", // build command
"start:cmd": "-Denvironment=cf -Dspring.profiles.active=provider,cloud -Xdebug -Xrunjdwp:server=y,transport=dt_socket,address=8000,suspend=n", // add profiles for tomcat start
"env": {} // add environment variables here
}
}
"start:sample-java": "cd scripts && node tomcat-adapter.js sample-java"
npm run start:sample-java
npm run start:<your_app_name>
> cloud-foundry-local-setup % npm run start:sample-java
> start:sample-java
> cd scripts && node tomcat-adapter.js sample-java
==> Maven Home /Users/i353584/.m2
==> App Name: sample-java
==> App Properties {
cfAppName: 'sample-java',
folder: './deps/sample-java',
path: 'sample-module/target',
artifactName: 'sample-java.war',
'build:cmd': 'mvn clean install',
'start:cmd': '-Denvironment=cf -Dspring.profiles.active=provider,cloud -Xdebug -Xrunjdwp:server=y,transport=dt_socket,address=8000,suspend=n',
env: {}
}
==> Building App - mvn clean install
[INFO] Scanning for projects...
[INFO] ------------------------------------------------------------------------
[INFO] Reactor Build Order:
[INFO]
[INFO] hello-spring-cloud [pom]
[INFO] sample-module [war]
[INFO]
*
*
*
[INFO] ------------------------------------------------------------------------
[INFO] Reactor Summary for hello-spring-cloud 0.0.1:
[INFO]
[INFO] hello-spring-cloud ................................. SUCCESS [ 3.549 s]
[INFO] sample-module ...................................... SUCCESS [ 4.866 s]
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
[INFO] Total time: 9.036 s
[INFO] Finished at: 2023-08-23T00:16:50+05:30
[INFO] ------------------------------------------------------------------------
==> setting vcap variables for mytrial - dev
==> using war file from ./deps/sample-java/sample-module/target/sample-java.war
Listening for transport dt_socket at address: 8000
*
*
*
SLF4J: Actual binding is of type [ch.qos.logback.classic.util.ContextSelectorStaticBinder]
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.7.9)
2023-08-22 18:46:58,653 [localhost-startStop-1] INFO com.sap.sample.ServletInitializer []:: Starting ServletInitializer v0.0.1 using Java 11.0.9 on ***** with PID 4726 (/Users/i353584/Documents/cloud-foundry-local-setup/deps/apache-tomcat-8.5.78/webapps/sample-java/WEB-INF/classes started by i353584 in /Users/i353584/Documents/cloud-foundry-local-setup/deps/apache-tomcat-8.5.78/bin)
2023-08-22 18:46:58,658 [localhost-startStop-1] INFO com.sap.sample.ServletInitializer []:: The following 2 profiles are active: "provider", "cloud"
2023-08-22 18:47:00,584 [localhost-startStop-1] INFO o.s.b.w.s.c.ServletWebServerApplicationContext []:: Root WebApplicationContext: initialization completed in 1824 ms
2023-08-22 18:47:01,530 [localhost-startStop-1] INFO o.s.s.web.DefaultSecurityFilterChain []:: Will secure any request with [org.springframework.security.web.session.DisableEncodeUrlFilter@39da0b6e, org.springframework.security.web.context.request.async.WebAsyncManagerIntegrationFilter@65d6c67a, org.springframework.security.web.context.SecurityContextPersistenceFilter@4f89d02, org.springframework.security.web.header.HeaderWriterFilter@2a6292f, org.springframework.security.web.csrf.CsrfFilter@5bc6d1bc, org.springframework.security.web.authentication.logout.LogoutFilter@6174885d, org.springframework.security.oauth2.server.resource.web.BearerTokenAuthenticationFilter@382648e6, org.springframework.security.web.savedrequest.RequestCacheAwareFilter@13c7f675, org.springframework.security.web.servletapi.SecurityContextHolderAwareRequestFilter@6a0d2b48, org.springframework.security.web.authentication.AnonymousAuthenticationFilter@6f3dd35e, org.springframework.security.web.session.SessionManagementFilter@6a749bc4, org.springframework.security.web.access.ExceptionTranslationFilter@16663510, org.springframework.security.web.access.intercept.FilterSecurityInterceptor@1516ca23]
2023-08-22 18:47:01,554 [localhost-startStop-1] WARN c.s.c.s.x.a.XsuaaAutoConfiguration []:: In productive environment provide a well configured client secret based RestOperations bean
2023-08-22 18:47:01,707 [localhost-startStop-1] INFO c.s.s.ApplicationStartOperationsHandler []:: Initial Start
2023-08-22 18:47:02,224 [localhost-startStop-1] INFO com.sap.sample.ServletInitializer []:: Started ServletInitializer in 4.913 seconds (JVM running for 10.893)
23-Aug-2023 00:17:02.261 INFO [localhost-startStop-1] org.apache.catalina.startup.HostConfig.deployWAR Deployment of web application archive [/Users/i353584/Documents/cloud-foundry-local-setup/deps/apache-tomcat-8.5.78/webapps/sample-java.war] has finished in [9,331] ms
23-Aug-2023 00:17:02.264 INFO [main] org.apache.coyote.AbstractProtocol.start Starting ProtocolHandler ["http-nio-8080"]
23-Aug-2023 00:17:02.279 INFO [main] org.apache.catalina.startup.Catalina.start Server startup in 9441 ms
Be aware that some ports might be blocked by other applications on your system. If you encounter this issue, follow the instructions below to change the ports:
- destinations.json # contains destinations to different back-end services.
- xs-app.json # contains routes, If route matches, forward to respective destination.
- pull-token.js # script to fetch bearer token of the user.
{
{
"name": "sample-java", // destination name
"url": "http://localhost:8080/sample-java", // url pointing to backing service
"forwardAuthToken": true, // send bearer token to backend.
"timeout": 6000000
}
}
{
"source": "^/sap/opu/rest/java/(.*)$",
"target": "$1",
"authenticationType": "xsuaa",
"destination": "sample-java"
}
npm run start:app-router
Prefer using a technical user for the app-router login, add the technical user in your space and org and provide access to it, then use it to login here.
> cloud-foundry-local-setup % npm run start:app-router
> start:app-router
> cd app-router && node index.js -p 9200
#2.0#2023 08 22 22:12:43:517#+05:30#WARNING#/LoggingLibrary################PLAIN##Dynamic log level switching not available#
[session-management] setting up session with identityzone : mytrial
prompt: Consumer Subdomain: (mytrial)
prompt: Email or UserId: TestTechincalUser
prompt: password:
[session-management] session created.
#2.0#2023 08 22 22:13:03:679#+05:30#INFO#/approuter#####llmjbkow##########llmjbkow#PLAIN##Application router version 10.15.4#
#2.0#2023 08 22 22:13:03:685#+05:30#INFO#/Configuration#####llmjbkp1##########llmjbkp1#PLAIN##No COOKIES environment variable#
#2.0#2023 08 22 22:13:03:691#+05:30#WARNING#/Configuration#####llmjbkp7##########llmjbkp7#PLAIN## Scopes defined in routes will be ignored.#
#2.0#2023 08 22 22:13:04:043#+05:30#INFO#/approuter#####llmjbkow##########llmjbkow#PLAIN##Application router is listening on port: 9200#
FLP Sandbox
<script>
window["sap-ushell-config"] = {
defaultRenderer: "fiori2",
bootstrapPlugins: {
"KeyUserPlugin": {
"component": "sap.ushell.plugins.rta"
},
"PersonalizePlugin": {
"component": "sap.ushell.plugins.rta-personalize"
}
},
applications: {
"sample-app": {
title: "Sample App", // tile name
description: "Sample App for Training",
additionalInformation: "SAPUI5.Component=com.sample.app", // app component id
applicationType: "URL",
url: "/resources/com/sap/sample-ui/", // route in xsapp.json to fetch the ui files
navigationMode: "embedded"
}
}
};
</script>
{
"source": "^/resources/com/sap/sample-ui/(.*)$",
"target": "$1",
"cacheControl": "no-cache",
"localDir": "../deps/sample-ui/sample-ui-html5/webapp"
}
{
"crossNavigation": {
"inbounds": {
"intent1": {
"signature": {
"parameters": {},
"additionalParameters": "allowed"
},
"semanticObject": "Sample",
"action": "display"
}
}
}
}
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
20 | |
11 | |
8 | |
8 | |
7 | |
7 | |
7 | |
6 | |
6 | |
6 |