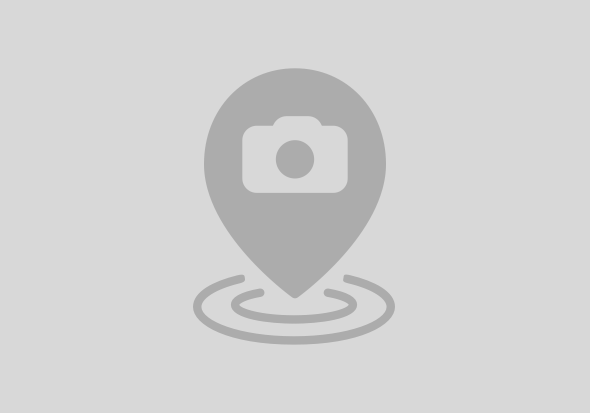
app.get('/', (req, res) => {
res.send('<p>Welcome to OPEN API Gateway</p><p>Service is Up & Running </p><p></p>' + Date());
})
app.listen(port, () => {
console.log(`Open AI App is listening on port ${port}`)
})
// Endpoint to add more data to the JSON file
app.post('/add', (req, res) => {
try {
//Fetch Vector
var newText = req.body.entry;
const session_url = 'https://api.openai.com/v1/embeddings';
var config_post = {
method: 'post',
url: session_url,
headers: {
'Authorization': 'Bearer '+token ,
'Content-Type' : 'application/json'
},
data: {
"model": "text-embedding-ada-002",
"input": newText
}
};
axios(config_post)
.then(function (response) {
const jsondata = readDataFromFile();
ques_vector = response.data["data"][0].embedding;
var len = jsondata.length;
//Format Data for Pushing
var id;
console.log(len);
if(len>0){
id = jsondata[len - 1].id + 1;
}else{
id = 0;
}
var entry = {
id : id,
text : newText,
embedding : ques_vector
}
//Push data to JSON
const existingData = readDataFromFile();
existingData.push(entry);
saveDataToFile(existingData);
res.status(201).json({ message: 'Data added successfully.' });
//End of Push Data
})
.catch(function (error) {
//Error
console.log(error);
res.send(error);
});
} catch (err) {
res.status(500).json({ error: 'Failed to add data.' });
}
});
// Helper function to read data from the JSON file
function readDataFromFile() {
try {
const data = fs.readFileSync(dataFilePath, 'utf8');
return JSON.parse(data);
} catch (err) {
// If the file doesn't exist or is empty, return an empty array
return [];
}
}
// Helper function to save data to the JSON file
function saveDataToFile(data) {
fs.writeFileSync(dataFilePath, JSON.stringify(data, null, 2), 'utf8');
}
// Endpoint to display the content of the JSON file
app.get('/display', (req, res) => {
try {
const data = readDataFromFile();
res.status(200).json(data);
} catch (err) {
res.status(500).json({ error: 'Failed to retrieve data.' });
}
});
// Endpoint to delete content from the JSON file
app.delete('/delete/:index', (req, res) => {
try {
const indexToDelete = parseInt(req.params.index);
const existingData = readDataFromFile();
if (indexToDelete < 0 || indexToDelete >= existingData.length) {
return res.status(400).json({ error: 'Invalid index.' });
}
existingData.splice(indexToDelete, 1);
saveDataToFile(existingData);
res.status(200).json({ message: 'Data deleted successfully.' });
} catch (err) {
res.status(500).json({ error: 'Failed to delete data.' });
}
});
// Call the Open AI
app.post('/askai', function (req, res) {
const data = readDataFromFile();
//var data = require('./data');
var ques = req.body.ques;
let table = [];
let tabrow = [];
let top3 = [];
//Fetch Vector
const session_url = 'https://api.openai.com/v1/embeddings';
var config_post = {
method: 'post',
url: session_url,
headers: {
'Authorization': 'Bearer '+token ,
'Content-Type' : 'application/json'
},
data: {
"model": "text-embedding-ada-002",
"input": ques
}
};
//Call
axios(config_post)
.then(function (response) {
ques_vector = response.data["data"][0].embedding;
var len = data.length;
//Check Similarities
for (let i = 0; i < len; i++) {
tabrow = [data[i].text, similarity(ques_vector, data[i].embedding)];
table.push(tabrow);
}
//Sort
table = table.sort((a, b) => a[1] - b[1]);
console.log("Question : "+ques);
console.log("Similarity Check");
//Sort Descending
console.log(table.reverse());
console.log("Top 3 Matches");
console.log(table[0]);
console.log(table[1]);
console.log(table[2]);
//Top 3
top3.push(table[0]);
top3.push(table[1]);
top3.push(table[2]);
res.send(top3);
})
.catch(function (error) {
//Error
console.log(error);
});
//
})
const express = require('express');
const bodyParser = require('body-parser');
const fs = require('fs');
const app = express();
var similarity = require( 'compute-cosine-similarity' );
const basicAuth = require('express-basic-auth');
var cors = require('cors');
const axios = require('axios');
const PORT = 8080;
const token = 'sk--[Your OpenAI Secret Key]--';
// Data File
const dataFilePath = 'data.json';
// Middleware to parse incoming JSON data & Disable CORS
app.use(bodyParser.json());
app.use(cors())
//Adding Auth
app.use(basicAuth({
users: { 'admin': 'oursecret' }
}))
// Welcome Page
app.get('/', (req, res) => {
res.send('<p>Welcome to OPEN API Gateway</p><p>Service is Up & Running </p><p></p>' + Date());
})
// Endpoint to add more data to the JSON file
app.post('/add', (req, res) => {
try {
//Fetch Vector
var newText = req.body.entry;
const session_url = 'https://api.openai.com/v1/embeddings';
var config_post = {
method: 'post',
url: session_url,
headers: {
'Authorization': 'Bearer '+token ,
'Content-Type' : 'application/json'
},
data: {
"model": "text-embedding-ada-002",
"input": newText
}
};
axios(config_post)
.then(function (response) {
const jsondata = readDataFromFile();
ques_vector = response.data["data"][0].embedding;
var len = jsondata.length;
//Format Data for Pushing
var id;
console.log(len);
if(len>0){
id = jsondata[len - 1].id + 1;
}else{
id = 0;
}
var entry = {
id : id,
text : newText,
embedding : ques_vector
}
//Push data to JSON
const existingData = readDataFromFile();
existingData.push(entry);
saveDataToFile(existingData);
res.status(201).json({ message: 'Data added successfully.' });
//End of Push Data
})
.catch(function (error) {
//Error
console.log(error);
res.send(error);
});
} catch (err) {
res.status(500).json({ error: 'Failed to add data.' });
}
});
// Endpoint to display the content of the JSON file
app.get('/display', (req, res) => {
try {
const data = readDataFromFile();
res.status(200).json(data);
} catch (err) {
res.status(500).json({ error: 'Failed to retrieve data.' });
}
});
// Endpoint to delete content from the JSON file
app.delete('/delete/:index', (req, res) => {
try {
const indexToDelete = parseInt(req.params.index);
const existingData = readDataFromFile();
if (indexToDelete < 0 || indexToDelete >= existingData.length) {
return res.status(400).json({ error: 'Invalid index.' });
}
existingData.splice(indexToDelete, 1);
saveDataToFile(existingData);
res.status(200).json({ message: 'Data deleted successfully.' });
} catch (err) {
res.status(500).json({ error: 'Failed to delete data.' });
}
});
// Call the Open AI
app.post('/askai', function (req, res) {
const data = readDataFromFile();
//var data = require('./data');
var ques = req.body.ques;
let table = [];
let tabrow = [];
let top3 = [];
//Fetch Vector
const session_url = 'https://api.openai.com/v1/embeddings';
var config_post = {
method: 'post',
url: session_url,
headers: {
'Authorization': 'Bearer '+token ,
'Content-Type' : 'application/json'
},
data: {
"model": "text-embedding-ada-002",
"input": ques
}
};
//Call
axios(config_post)
.then(function (response) {
ques_vector = response.data["data"][0].embedding;
var len = data.length;
//Check Similarities
for (let i = 0; i < len; i++) {
tabrow = [data[i].text, similarity(ques_vector, data[i].embedding)];
table.push(tabrow);
}
//Sort
table = table.sort((a, b) => a[1] - b[1]);
console.log("Question : "+ques);
console.log("Similarity Check");
//Sort Descending
console.log(table.reverse());
console.log("Top 3 Matches");
console.log(table[0]);
console.log(table[1]);
console.log(table[2]);
//Top 3
top3.push(table[0]);
top3.push(table[1]);
top3.push(table[2]);
res.send(top3);
})
.catch(function (error) {
//Error
console.log(error);
});
//
})
// Helper function to read data from the JSON file
function readDataFromFile() {
try {
const data = fs.readFileSync(dataFilePath, 'utf8');
return JSON.parse(data);
} catch (err) {
// If the file doesn't exist or is empty, return an empty array
return [];
}
}
// Helper function to save data to the JSON file
function saveDataToFile(data) {
fs.writeFileSync(dataFilePath, JSON.stringify(data, null, 2), 'utf8');
}
// Endpoint to add more data to the JSON file
app.post('/add', (req, res) => {
try {
//Fetch Vector
var newText = req.body.entry;
const session_url = 'https://api.openai.com/v1/embeddings';
var config_post = {
method: 'post',
url: session_url,
headers: {
'Authorization': 'Bearer '+token ,
'Content-Type' : 'application/json'
},
data: {
"model": "text-embedding-ada-002",
"input": newText
}
};
axios(config_post)
.then(function (response) {
const jsondata = readDataFromFile();
ques_vector = response.data["data"][0].embedding;
var len = jsondata.length;
//Format Data for Pushing
console.log(len);
console.log(jsondata[len - 1].id);
var entry = {
id : jsondata[len - 1].id + 1,
text : newText,
embedding : ques_vector
}
//Push data to JSON
const existingData = readDataFromFile();
existingData.push(entry);
saveDataToFile(existingData);
res.status(201).json({ message: 'Data added successfully.' });
//End of Push Data
})
.catch(function (error) {
//Error
console.log(error);
res.send(error);
});
} catch (err) {
res.status(500).json({ error: 'Failed to add data.' });
}
});
// Endpoint to display the content of the JSON file
app.get('/display', (req, res) => {
try {
const data = readDataFromFile();
res.status(200).json(data);
} catch (err) {
res.status(500).json({ error: 'Failed to retrieve data.' });
}
});
// Endpoint to delete content from the JSON file
app.delete('/delete/:index', (req, res) => {
try {
const indexToDelete = parseInt(req.params.index);
const existingData = readDataFromFile();
if (indexToDelete < 0 || indexToDelete >= existingData.length) {
return res.status(400).json({ error: 'Invalid index.' });
}
existingData.splice(indexToDelete, 1);
saveDataToFile(existingData);
res.status(200).json({ message: 'Data deleted successfully.' });
} catch (err) {
res.status(500).json({ error: 'Failed to delete data.' });
}
});
// Call the Open AI
app.post('/askai', function (req, res) {
const data = readDataFromFile();
//var data = require('./data');
var ques = req.body.ques;
let table = [];
let tabrow = [];
let top3 = [];
//Fetch Vector
const session_url = 'https://api.openai.com/v1/embeddings';
var config_post = {
method: 'post',
url: session_url,
headers: {
'Authorization': 'Bearer '+token ,
'Content-Type' : 'application/json'
},
data: {
"model": "text-embedding-ada-002",
"input": ques
}
};
//Call
axios(config_post)
.then(function (response) {
ques_vector = response.data["data"][0].embedding;
var len = data.length;
//Check Similarities
for (let i = 0; i < len; i++) {
tabrow = [data[i].text, similarity(ques_vector, data[i].embedding)];
table.push(tabrow);
}
//Sort
table = table.sort((a, b) => a[1] - b[1]);
console.log("Question : "+ques);
console.log("Similarity Check");
//Sort Descending
console.log(table.reverse());
console.log("Top 3 Matches");
console.log(table[0]);
console.log(table[1]);
console.log(table[2]);
//Top 3
top3.push(table[0]);
top3.push(table[1]);
top3.push(table[2]);
res.send(top3);
})
.catch(function (error) {
//Error
console.log(error);
});
//
})
// Helper function to read data from the JSON file
function readDataFromFile() {
try {
const data = fs.readFileSync(dataFilePath, 'utf8');
return JSON.parse(data);
} catch (err) {
// If the file doesn't exist or is empty, return an empty array
return [];
}
}
// Helper function to save data to the JSON file
function saveDataToFile(data) {
fs.writeFileSync(dataFilePath, JSON.stringify(data, null, 2), 'utf8');
}
// Start the server
app.listen(PORT, () => {
console.log(`OpenAI API is running on port ${PORT}`)
})
npm start
http://localhost:8080/add
{
"entry":"Need Help"
}
{
"entry":"Text/Phrase to be Trained"
}
Travel, Finance, IT Support
http://localhost:8080/diplay
http://localhost:8080/askai
{
"ques":"My Laptop isn't working"
}
{
"ques":"/Your Query/"
}
http://localhost:8080/delete/2
http://localhost:8080/diplay
You must be a registered user to add a comment. If you've already registered, sign in. Otherwise, register and sign in.
User | Count |
---|---|
6 | |
5 | |
5 | |
4 | |
4 | |
4 | |
3 | |
3 | |
3 | |
3 |